In this post, Creating a responsive sidebar for your website can significantly enhance user experience and navigation. With the rise of mobile devices, having a sidebar that adapts to different screen sizes is crucial. In this article, we will explore how to create a responsive sidebar using ReactJS and TailwindCSS
Why Use ReactJS and TailwindCSS?
ReactJS is a JavaScript library that allows you to build user interfaces for web applications. It’s known for its efficiency and flexibility, making it an excellent choice for creating dynamic and interactive components like sidebars. TailwindCSS, on the other hand, is a utility-first CSS framework that helps you quickly build custom designs without writing any CSS.
Setting Up Your Project
Before we dive into creating the sidebar, make sure you have Node.js installed on your machine. You can check if Node.js is installed by running the following command in your terminal:
If Node.js is not installed, you can download it from the official website and follow the installation instructions. Once you have Node.js installed, you can create a new React project using Vitejs
Note!
After downloading the template, please run the following commands:
Integrating the Sidebar into Your App
To integrate the sidebar into your app, you need to import the Sidebar
component into your App.js
file and add it to your app’s layout:
import { useState } from 'react'
import Sidebar from './container/Sidebar'
function App() {
return (
<div className='flex'>
<Sidebar/>
</div>
)
}
export default App
JavaScriptCreating the Sidebar Component
Now that your project is set up, you can start creating the sidebar component. In the src directory, create a new file called Sidebar.js and add the following code:
import React from 'react'
import logo from '../assets/images/logo.png'
import { IoIosLogOut } from "react-icons/io";
import { PiWalletDuotone } from "react-icons/pi";
import { IoMdHome } from "react-icons/io";
import { FaChartLine } from "react-icons/fa";
import { MdPerson } from "react-icons/md";
const Sidebar = () => {
const [SidebarData, setSidebarData] = React.useState([
{
id: 1,
name: 'Home',
icon: <IoMdHome />,
active: true
}
,
{
id: 2,
name: 'Chart',
icon: <FaChartLine />,
active: false
}
,
{
id: 3,
name: 'Person',
icon: <MdPerson />,
active: false
}
])
const handleItemClick = (id) => {
const updatedData = SidebarData.map(item => {
if (item.id === id) {
return { ...item, active: true };
} else {
return { ...item, active: false };
}
});
setSidebarData(updatedData);
};
return (
<section>
<div className='w-[100px] text-white bg-[--bg-secondary] h-screen flex-col justify-between shadow-lg sm:flex hidden'>
<div className=' mx-auto mt-10 text-4xl '>
<PiWalletDuotone />
</div>
<div className='text-4xl relative w-full flex flex-col mx-auto'>
{
SidebarData.map((item, index) => (
<div
key={index}
className={` items-center justify-center flex w-full mb-20 ${item.active ? 'active' : 'icons'}`}
onClick={() => handleItemClick(item.id)}
>
{item.icon}
</div>
))
}
</div>
<div
className='text-4xl mx-auto mb-20'
><IoIosLogOut className='icon' /></div>
</div>
{/* Responsive */}
<div className='max-h-[70px] p-3 absolute bottom-0 text-white bg-[--bg-secondary] w-screen justify-between shadow-lg sm:hidden flex'>
<div className=' mx-auto text-4xl '>
<PiWalletDuotone />
</div>
<div className='text-4xl relative w-full flex mx-auto'>
{
SidebarData.map((item, index) => (
<div
key={index}
className={`mx-auto icons mb-20 ${item.active ? 'active1' : ''}`}
onClick={() => handleItemClick(item.id)}
>
{item.icon}
</div>
))
}
</div>
<div
className='text-4xl mx-auto mb-20 '
><IoIosLogOut className='icon' /></div>
</div>
</section>
)
}
export default Sidebar
JavaScriptIn this code, we have created a basic sidebar component with links to different sections of your website. We have used TailwindCSS classes to style the sidebar and make it responsive.
Now, when you run your React app using npm start
, you should see the responsive sidebar on the left side of your app’s layout. You can customize the sidebar’s appearance and content to suit your needs.
Add custom CSS file
Certainly! You can add custom CSS to further style your sidebar. Here’s an example of how you can do it:
In your Sidebar.js
file, you can add a style
object and apply it to your sidebar div:
@tailwind base;
@tailwind components;
@tailwind utilities;
:root{
--bg-primary: #191b20;
--bg-secondary: #23272f;
}
body{
background-color: var(--bg-primary);
color: white;
}
.active::before{
position: absolute;
content: '';
width: 3px;
height: 50px;
background-color: white;
right: -2px;
z-index: -1;
box-shadow: 0 0 10px 0px white;
border-radius: 10px;
transition: all 0.5s ease;
}
.active{
background: transparent;
color: white;
filter: drop-shadow(1px 2px 10px rgba(255, 255, 255, 0.649));
transition: all 0.5s ease;
}
.icons{
color: #7a7a81;
}
.icons:hover,.icon:hover{
filter: drop-shadow(1px 2px 10px rgba(255, 255, 255, 0.649));
transition: all 0.3s ease;
}
.active1{
color: white;
padding: 0px 10px 0px 0px;
filter: drop-shadow(1px 2px 10px rgba(255, 255, 255, 0.649));
transition: all 0.3s ease;
}
.active1::before{
position: absolute;
content: '';
width: 100%;
height: 3px;
background-color: white;
top: -12px;
box-shadow: 0 0 10px 0px white;
border-radius: 10px;
}
.icon{
color: white;
font-weight: bolder;
}
CSSOUTPUT:
If you found this blog post helpful, please consider sharing it with others who might benefit. You can also follow me for more content on Javascript, React, and other web Development topics.
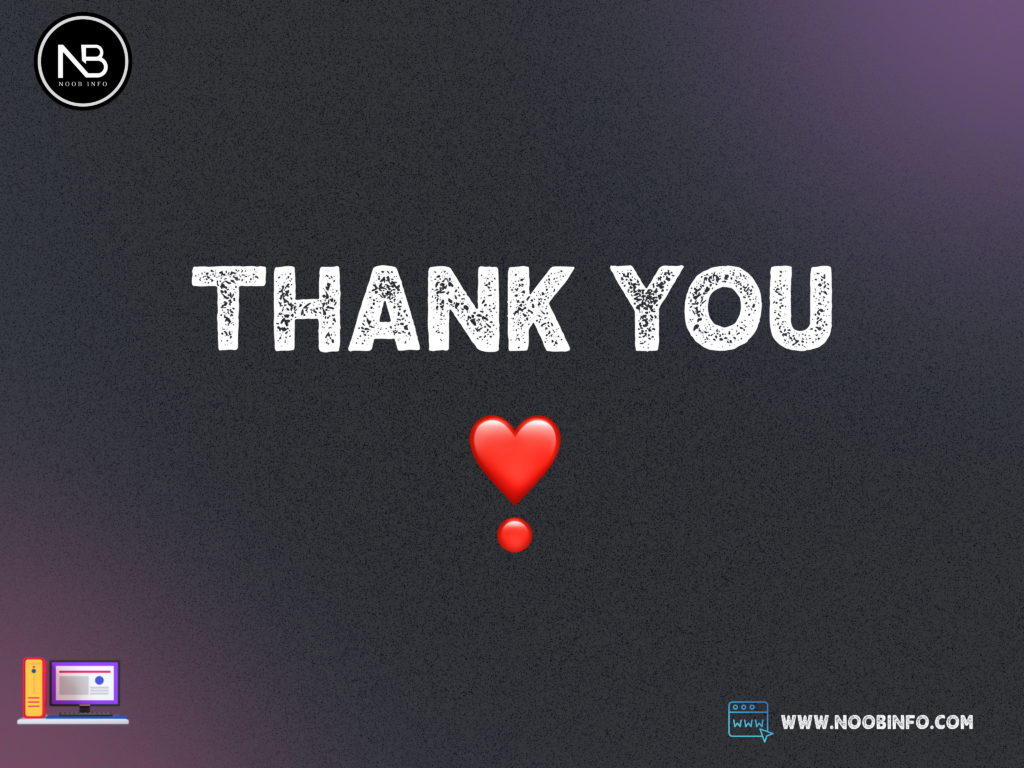
Generally I do not read article on blogs, but I wish to
say that this write-up very forced me to try and do so!
Your writing style has been surprised me. Thanks, very nice
post.
Thanks for your Support❣️❣️